How to Integrate Share on Omnia on your Platform
Here is the step by step guide for integrating Share on Omnia onto your platform that allows your users to seamlessly share content with their on Omnia network. Follow the steps below to integrate Share on Omnia functionality into your web pages.
- Step 1: Go to the Account Settings by clicking on the profile tab and click on the Integration Keys tab.
- Step 2: Generate client id and client secret by clicking the ‘Generate’ button.
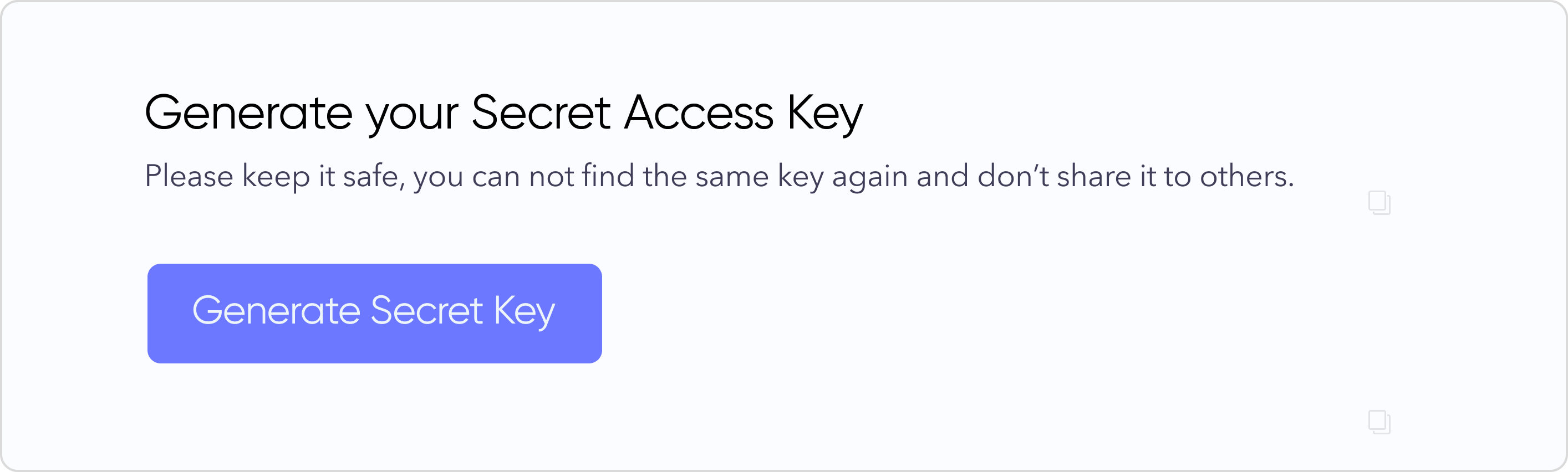
Please keep it safe, you can not find the same key again and don't share it to others.
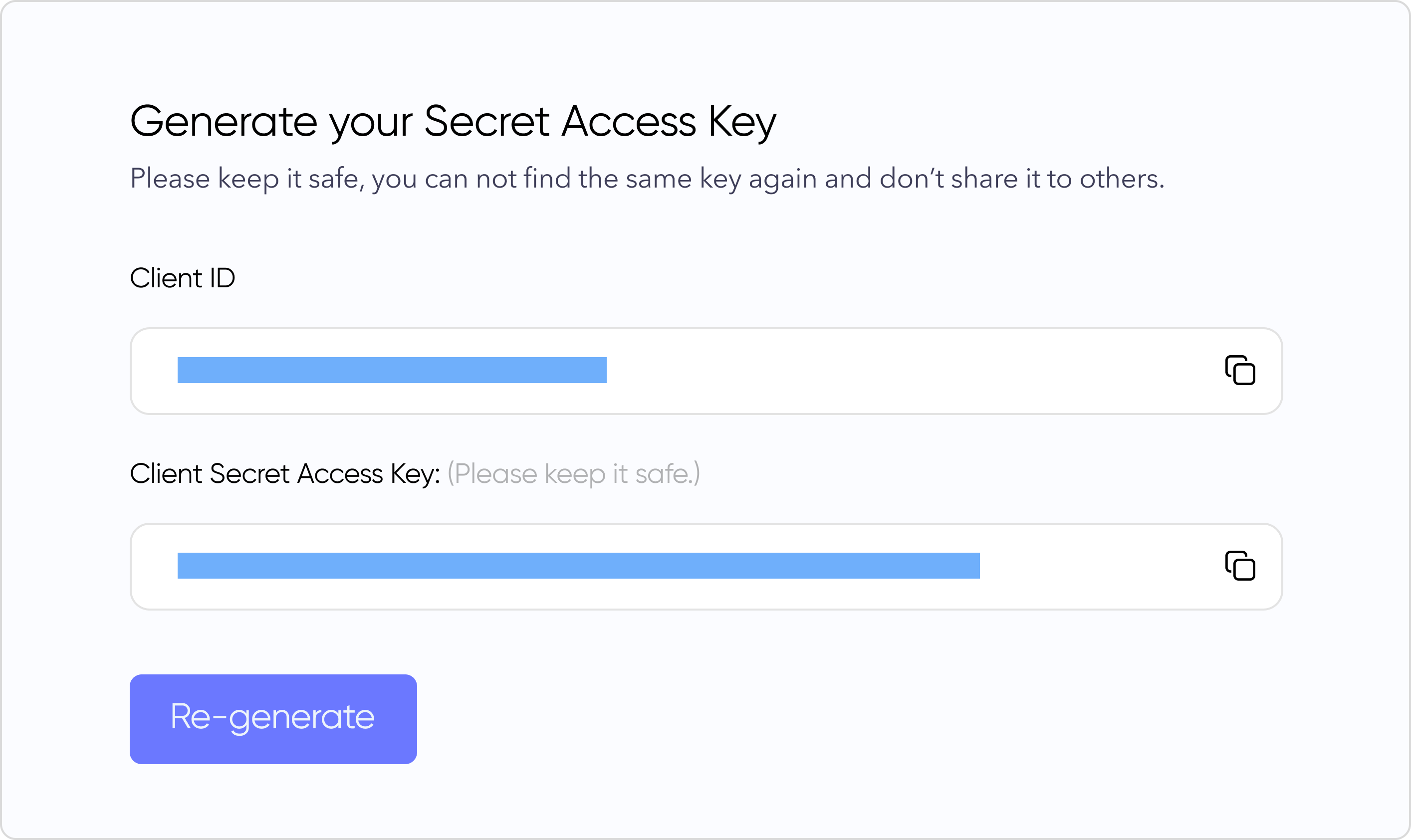
Make sure to copy the client secret somewhere (preferably .env) before closing this page. You will not able to see it again!
You can again regenerate a new client secret. This will make your old key invalid
The client secret must be kept secure. Do not share it publicly. The Omnia team will never ask you for your client secret.
- Step 3: Enter the domain(s) those will be origin of your request.
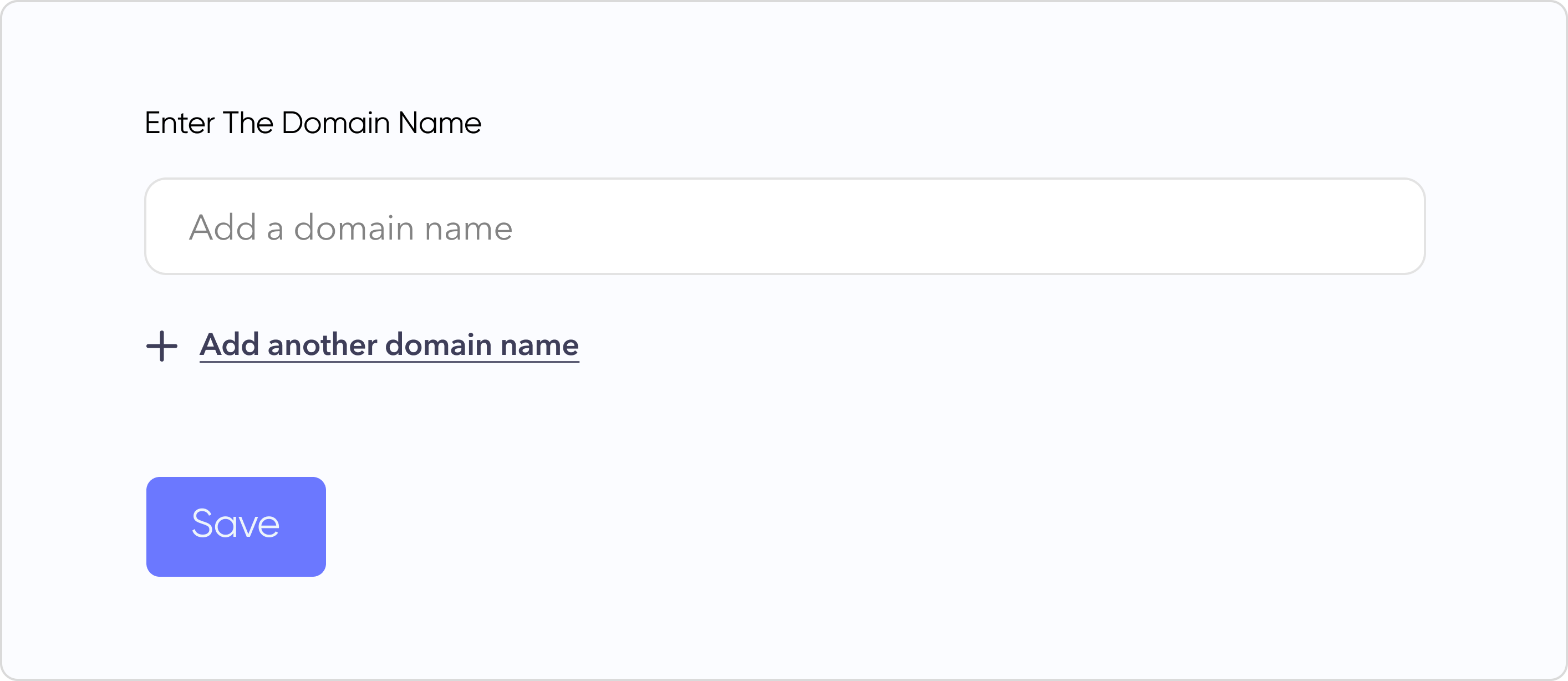
You can add domain more than one, the site where you want to integrate the Omnia.
- Step 4: Add the script tag in your html head tag in your code.
<script src=`https://www.theomnia.io/api/sdk/get-omnia-sdk/${your_client_id}`></script>
Replace ${your_client_id} with the client id you generated from Integration keys tab and paste into html head tag of your code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
//Share on Omnia script
<script src=`https://www.theomnia.io/api/sdk/get-omnia-sdk/12345678-abcd-efgh-1234567890ab`></script>
<title>Document</title>
</head>
<body>
</body>
</html>
- Step 5: Create your backend api to call Omnia createShare api.
Endpoint: https://theomnia.io/api/sdk/omnia-sdk
Authentication: Ensure to include the Authorization header with a Bearer token.
Credentials: Include credentials in the request to verify your identity.
Request Method: GET
Request Headers:
Authorization: Bearer `your_access_token`
Content-Type: application/json
Credential: include
The createShare function verifies your identity and sets up the details of the share. Pass createShare as a parameter in "Omnia.Share". When the buyer selects your "Share" button, createOrder launches the Omnia share window. The user logs in and shares their post on the theomnia.io website.
Replace ${YOUR_CLIENT_SECRET} with the client secret you generated from Integration keys tab.
app.post("/my-server/create-omnia-share", async (req, res) => {
const order = await createOrder();
res.json(order);
});
// use the omnia api to initiate a share
function createShare() {
// Add the generated client secret
// make a "GET" request with with your client secret
// make sure to add credentials request. This helps us to verify your identity
const accessToken = "YOUR_CLIENT_SECRET";
return fetch("https://theomnia.io/api/sdk/omnia-sdk", {
method: "GET",
credentials: 'include',
headers: {
"Content-Type": "application/json",
Authorization: `Bearer ${accessToken}`,
},
}).then((response) => response.json());
}
- Step 6: Create your backend api to call Omnia createShare api.
From front-end, create a function that calls your /my-server/create-omnia-share api. Pass this function as a parameter to "Omnia.share"
Create a button and call the Omnia.Share function on click of the button.
Pass the authenticated user's email and createShare function to the "Omnia.Share" function as parameter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Share on Omnia</title>
</head>
<body>
<div class="App">
<button id="share-on-omnia-button" type="button">
Share on Omnia
</button>
</div>
<script>
document.getElementById("share-on-omnia-button").addEventListener("click", function () {
const email = "user@example.com"; // Example email, replace with actual logic to get email
Omnia.Share({
email, // required
createShare, // required
});
});
async function createShare() {
try {
const response = await fetch("/my-server/create-omnia-share", {
method: "GET",
credentials: "include",
headers: { "Content-Type": "application/json" }
});
return response;
} catch (error) {
console.error(error);
throw error;
}
}
</script>
</body>
</html>
- Step 7: Optionally u can pass onSuccess and onError parameters to "Omnia.Share".
Omnia.Share accepts two more functions, onSuccess and onError. You can handle the sucess and error of the functionality to improve your user experience
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Share on Omnia</title>
</head>
<body>
<div class="App">
<button id="share-on-omnia-button" type="button">
Share on Omnia
</button>
</div>
<script>
async function createShare() {
//logic to create a share
}
function onSuccess() {
// Handle success logic
console.log("Share was successful");
}
function onError(error) {
// Handle error logic
console.error("Error creating share:", error);
}
document.getElementById("share-on-omnia-button").addEventListener("click", function () {
const email = "user@example.com"; // Example email, replace with actual logic to get email
Omnia.Share({
email, // required
createShare, // required
onSuccess, // Optional
onError, // Optional
});
});
</script>
</body>
</html>
This documentation provides a clear and concise guide on how to interact with the Omnia SDK endpoint using JavaScript. It covers authentication requirements, request method, headers, and includes a practical example to help integrate the Omnia API into your application effectively.
You might also like
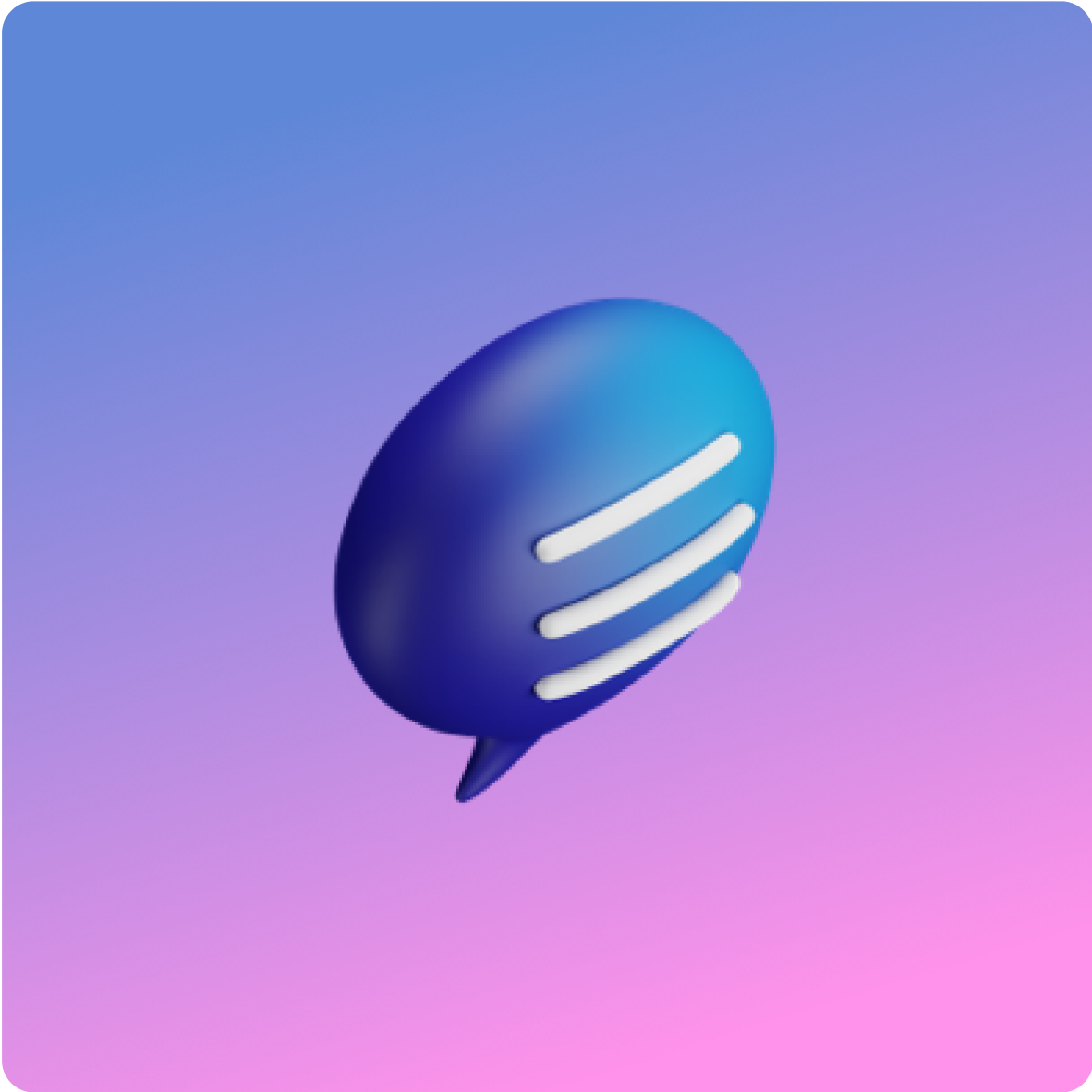
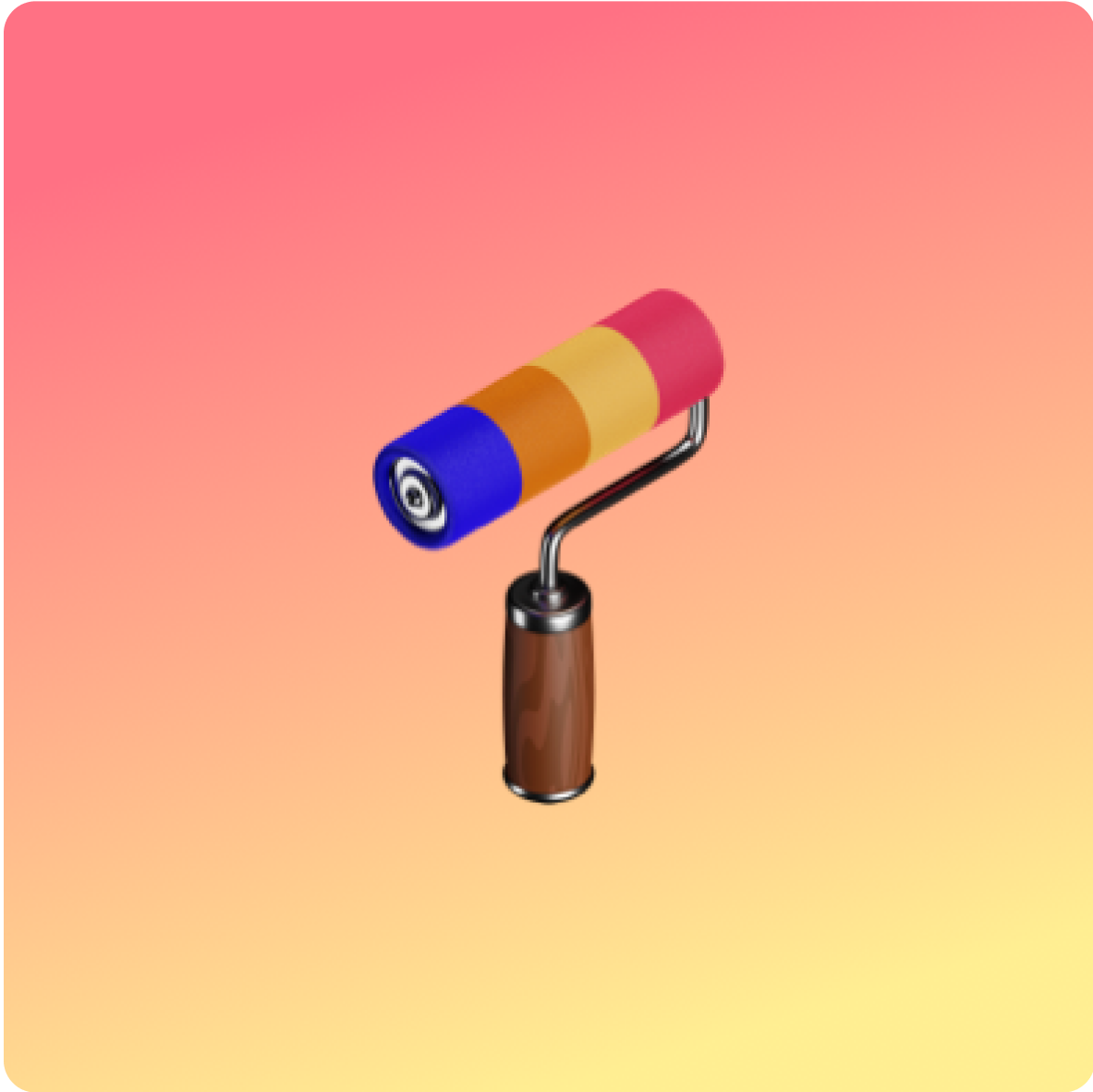
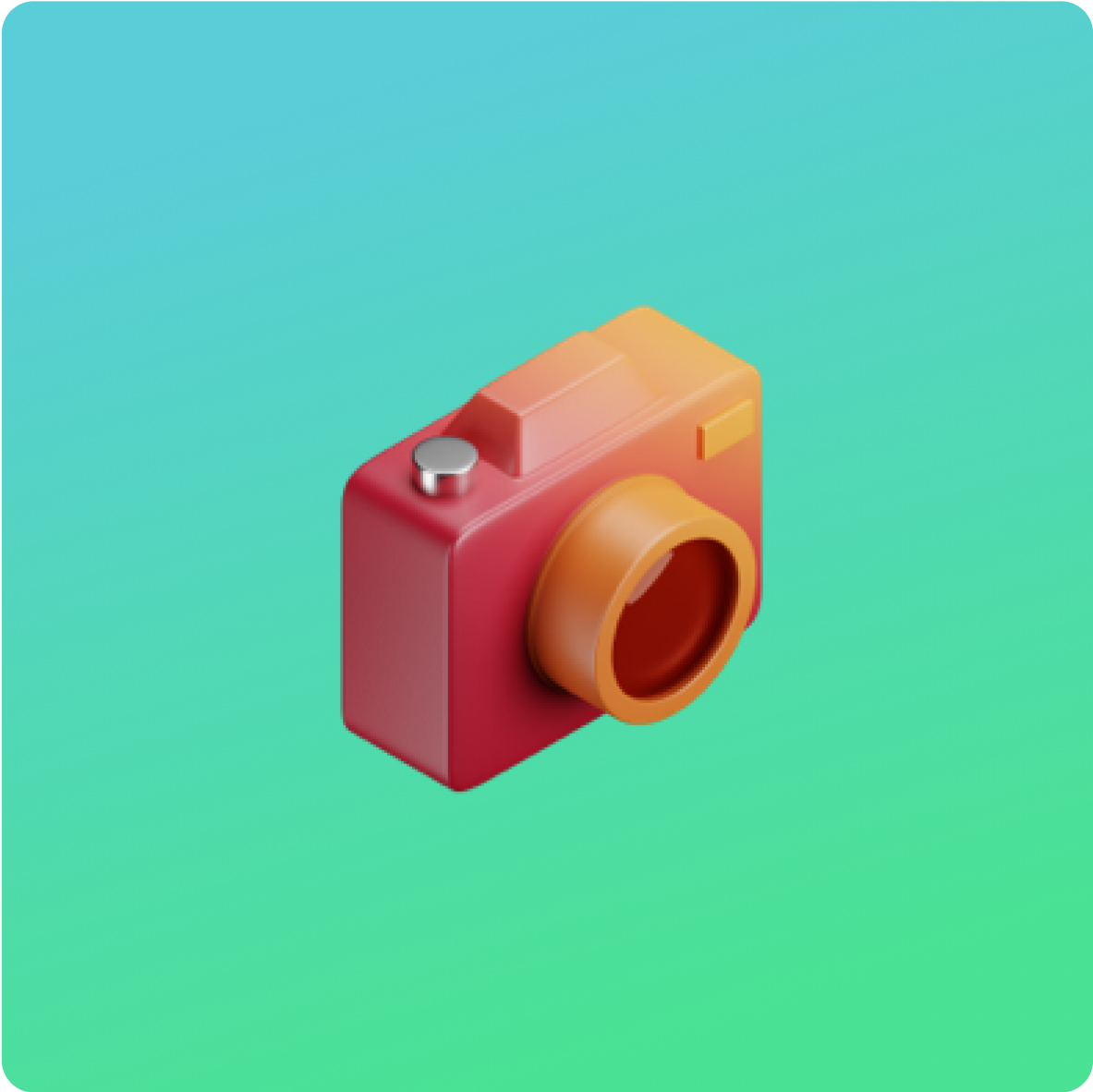